Prompt Chaining
In this example, we’ll build a blog content generation Workflow that creates engaging, targeted content through a series of prompts. The Workflow takes an industry and target audience as inputs and produces a complete blog post through the following steps:
TopicResearch
: Generates and rates potential blog topics based on audience relevance and SEO potentialOutlineCreation
: Creates a detailed outline for the highest-rated topicContentGeneration
: Writes a complete blog post following the outlineFinalOutput
: Returns the final blog content
Which corresponds to a Workflow graph like this:
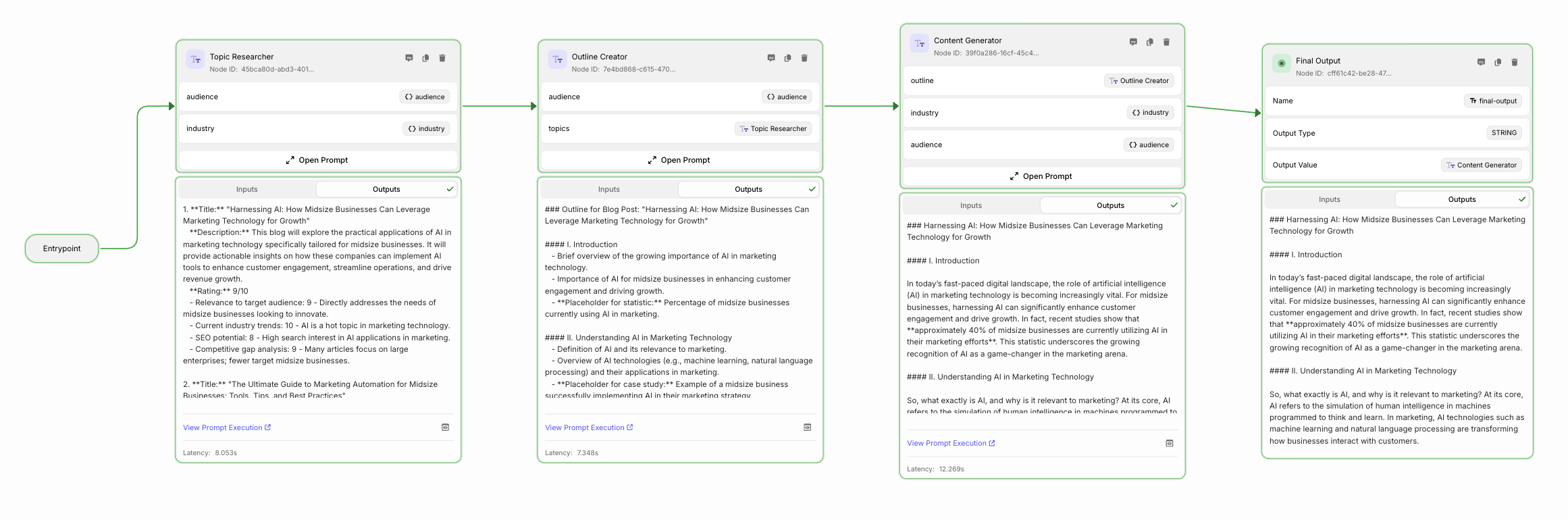
Setup
Install Vellum
Create your Project
Structure your project like this:
Define Workflow Inputs
The Workflow takes two inputs: the target audience and industry. These will be used to generate relevant, targeted content throughout the chain.
Build the Nodes
Topic Research
This node generates and rates potential blog topics based on the specified industry and audience.
Instantiate the Graph and Invoke it
Conclusion
We’ve built a prompt chain Workflow that generates blog content through a series of specialized prompts. Each node in the chain builds upon the previous one’s output, resulting in high-quality, targeted content. From here, you can:
- Version control the Workflow with your codebase
- Continue development in the Vellum UI
- Deploy to Vellum
- Add evaluation metrics to assess content quality
- Expand the chain with additional steps like SEO optimization or image generation